#1. Hi, I am going to continue from my previous post to talk about another way to style JSX component elements. In the previous post, I showed you how we can style the elements using the inline style syntax, but this time i will show you how to style with classes. So we had our output which looks like this:
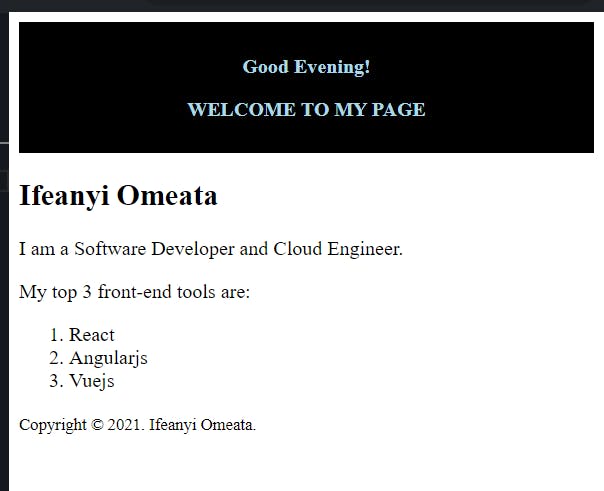
#2. We also had our Parent component 'App.js' file:
import React from 'react';
import MyHeader from './components/MyHeader';
import Main from './components/Main';
import MyFooter from './components/MyFooter';
function App() {
return (
<div>
<MyHeader />
<Main />
<MyFooter />
</div>
);
}
export default App;
#3. Next, we also had our child JSX components: Main.js, MyHeader.js and MyFooter.js:
Main.js:
import React from 'react';
function Main() {
return (
<div>
<h2>Ifeanyi Omeata</h2>
<p>I am a Software Developer and Cloud Engineer.</p>
<p>My top 3 front-end tools are:</p>
<ol>
<li>React</li>
<li>Angularjs</li>
<li>Vuejs</li>
</ol>
</div>
);
}
export default Main;
MyHeader.js:
import React from 'react';
const myHeaderStyle = {
backgroundColor: '#000',
color: '#fff',
fontWeight: 'bold',
padding: '10px',
textAlign: 'center',
};
let timeOfTheDay = 'Morning';
const date = new Date();
const hours = date.getHours();
if (hours < 12) {
timeOfTheDay = 'Morning';
myHeaderStyle.color = 'lightyellow';
} else if (hours < 17) {
timeOfTheDay = 'Afternoon';
myHeaderStyle.color = 'red';
} else {
timeOfTheDay = 'Evening';
myHeaderStyle.color = 'lightblue';
}
console.log(hours);
function MyHeader() {
return (
<header style={myHeaderStyle}>
<p>Good {timeOfTheDay}!</p>
<p>WELCOME TO MY PAGE</p>
</header>
);
}
export default MyHeader;
MyFooter.js:
import React from 'react';
function MyFooter() {
return <small>Copyright © 2021. Ifeanyi Omeata.</small>;
}
export default MyFooter;
#4. Next, we would replace the inline styling in the header tag, with a class based styling which would be a variable because of our if-statement. We need to get rid of the javascript method of changing the color directly in the if-statement; Instead, we would create 3 css styling options for the times of the day to replace that.
This is the initial:
This is the final adjustment:
import React from 'react';
let timeOfTheDay;
const mystyle = 'mystyle';
const date = new Date();
const hours = date.getHours();
if (hours < 12) {
timeOfTheDay = 'morning';
} else if (hours < 17) {
timeOfTheDay = 'afternoon';
} else {
timeOfTheDay = 'evening';
}
console.log(hours, timeOfTheDay);
function MyHeader() {
return (
<header className={timeOfTheDay}>
<p>Good {timeOfTheDay}!</p>
<p>WELCOME TO MY PAGE</p>
</header>
);
}
export default MyHeader;
This is what the style.css file will look like:
.morning {
background-color: #000;
font-weight: bold;
padding: 10px;
text-align: center;
color: yellow;
/* color: lightyellow; */
}
.afternoon {
background-color: #000;
font-weight: bold;
padding: 10px;
text-align: center;
color: red;
}
.evening {
background-color: #000;
font-weight: bold;
padding: 10px;
text-align: center;
color: blue;
}
#5. Finally, remember to import the style.css file into our index.js file:
import React from 'react';
import ReactDOM from 'react-dom';
import './style.css';
import App from './App';
ReactDOM.render(<App />, document.getElementById('root'));
#6. The final output should look like this. At the time of writing this post the time was 10:06am, hence we have the hours output as '10' and the timeOfTheDay output as 'morning'.
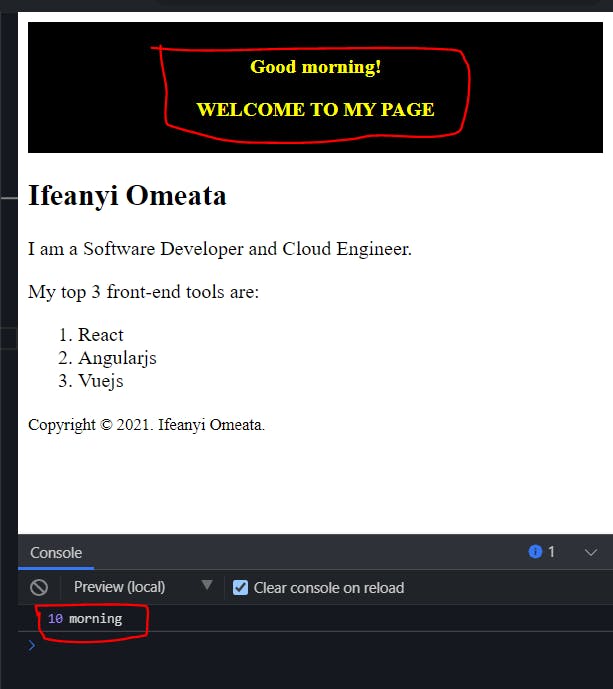
#End
Hope you enjoyed this! :) Follow me for more contents...
Get in Touch:
ifeanyiomeata.com
contact@ifeanyiomeata.com
Youtube: youtube.com/c/IfeanyiOmeata
Linkedin: linkedin.com/in/omeatai
Twitter: twitter.com/iomeata
Github: github.com/omeatai
Stackoverflow: stackoverflow.com/users/2689166/omeatai
Hashnode: hashnode.com/@omeatai
Medium: medium.com/@omeatai
© 2021