Tasks -
Task 1 Task 2 Task 3 Task 4 Task 5 Task 6 Task 7 Task 8 Task 9 Task 10 Task 11 Task 12 Task 13 Task 14 Task 15 Task 16 Task 17 Task 18 Task 19 Task 20
Task 1 -
I. Given an integer, write a program to display the factorial of that number.
II. Your program should display the output in the format:
"The factorial of (integer) is (factorial)."
III. For Example: Given 4, your program should display, “The factorial of 4 is 24.”
>>Return to Menu
Method I:
Task 2 -
I. Write a program to check if a given year is a leap year.
II. It should display True if it is a leap year and False if otherwise.
III. A leap year is one that is exactly divisible by 4 except for century years (ending with "00").
IV. A century year is a leap year only if it is perfectly divisible by 400.
V. Given 2000, your program should display True.
>>Return to Menu
Task 3 -
I. Write a program to draw an inverted triangle using the asterisks character.
II. Given a number of rows, the triangle would have 1 less than the given number of rows.
III. For Example: Given 6, your triangle would look like the below, an inverted triangle with 5 rows:
>>Return to Menu
Task 4 -
I. Write a program to draw a triangle using the Hash character.
II. Given a number of rows, the triangle would have 1 less than the given number of rows.
III. For Example: Given 6, your triangle would look like the below, a triangle with 5 rows:
>>Return to Menu
Task 5 -
I. Given an integer, write a program to check if the number is prime.
II. Note: A prime number is one divisible by 1 and itself.
III. If the number is prime: display - “{number} is a prime number".
IV. If the number is not prime: display - “{number} is not a prime number" and a list of its factors in a new line.
V. Example:
Hope you enjoyed this! :) Follow me for more contents...
Get in Touch:
ifeanyiomeata.com
contact@ifeanyiomeata.com
Task 1 Task 2 Task 3 Task 4 Task 5 Task 6 Task 7 Task 8 Task 9 Task 10 Task 11 Task 12 Task 13 Task 14 Task 15 Task 16 Task 17 Task 18 Task 19 Task 20
Task 1 -
I. Given an integer, write a program to display the factorial of that number.
II. Your program should display the output in the format:
"The factorial of (integer) is (factorial)."
III. For Example: Given 4, your program should display, “The factorial of 4 is 24.”
>>Return to Menu
Method I:
#Function to find factorial of num:
def factorial_of_num(num):
#Set init factorial value to 1.
#Loop through values of factors and multiply them together
factorial = 1
for factors in range(1,int(num)+1):
factorial *= factors
return f"The factorial of {int(num)} is {factorial}."
print(factorial_of_num(4))
Method II:
# Task 1 -
# I. Given an integer, write a program to display the factorial of that number.
# II. Your program should display the output in the format:
# "The factorial of (integer) is (factorial)."
# III. For Example: Given 4, your program should display, “The factorial of 4 is 24.”
import math
def factorial_of_num(num):
return f"The factorial of {int(num)} is {math.factorial(int(num))}."
print(factorial_of_num(4))
Task 2 -
I. Write a program to check if a given year is a leap year.
II. It should display True if it is a leap year and False if otherwise.
III. A leap year is one that is exactly divisible by 4 except for century years (ending with "00").
IV. A century year is a leap year only if it is perfectly divisible by 400.
V. Given 2000, your program should display True.
>>Return to Menu
# Task 2 -
# I. Write a program to check if a given year is a leap year.
# II. It should display True if it is a leap year and False if otherwise.
# III. A leap year is one that is exactly divisible by 4 except for century years (ending with "00").
# IV. A century year is a leap year only if it is perfectly divisible by 400.
# V. Given 2000, your program should display True.
def is_leap_year(the_year):
#If divisible by 4 but not a century year, then True
if(the_year % 4 == 0 and the_year % 100 != 0):
return True
#Else if a century year but divisible by 400, then True
elif(the_year % 100 == 0 and the_year % 400 == 0):
return True
#Else if neither condition holds, then False
else:
return False
print(is_leap_year(2000))
Task 3 -
I. Write a program to draw an inverted triangle using the asterisks character.
II. Given a number of rows, the triangle would have 1 less than the given number of rows.
III. For Example: Given 6, your triangle would look like the below, an inverted triangle with 5 rows:
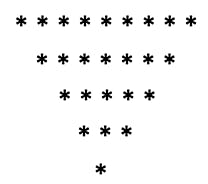
# Task 3 -
# I. Write a program to draw an inverted triangle using the asterisks character.
# II. Given a number of rows, the triangle would have 1 less than the given number of rows.
# III. For Example: Given 6, your triangle would look like the below, an inverted triangle with 5 rows.
#Function to draw inverted triangle
def inverted_triangle(num):
#Loop to create the rows using for loop in range
for x in range (num-1,0,-1):
#Develop the pattern for arranging the * symbols
print(" "*(num -x)+"* "*(2*x - 1))
inverted_triangle(6)
Task 4 -
I. Write a program to draw a triangle using the Hash character.
II. Given a number of rows, the triangle would have 1 less than the given number of rows.
III. For Example: Given 6, your triangle would look like the below, a triangle with 5 rows:
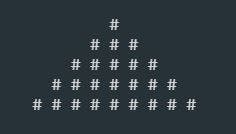
# Task 4 -
# I. Write a program to draw a triangle using the Hash character.
# II. Given a number of rows, the triangle would have 1 less than the given number of rows.
# III. For Example: Given 6, your triangle would look like the below, a triangle with 5 rows:
def triangle(num):
for x in range(1,num):
print((num-x) * " " + "# " * (2*x - 1))
triangle(6)
Task 5 -
I. Given an integer, write a program to check if the number is prime.
II. Note: A prime number is one divisible by 1 and itself.
III. If the number is prime: display - “{number} is a prime number".
IV. If the number is not prime: display - “{number} is not a prime number" and a list of its factors in a new line.
V. Example:
- Given 4, your program should display: “4 is not a prime number." [1,2,4]
- Given 7, your program should display: “7 is a prime number."
def is_prime_number(num):
#check is_valid
if num < 2 :
return f"{num} is not a prime number. {[num]}"
prime_list = [1]
for n in range(2,num):
if num % n == 0 :
prime_list.append(n)
if prime_list == [1]:
return f"{num} is a prime number."
else:
return f"{num} is not a prime number. {prime_list}"
print(is_prime_number(6))
#End
Hope you enjoyed this! :) Follow me for more contents...
Get in Touch:
ifeanyiomeata.com
contact@ifeanyiomeata.com
Youtube: youtube.com/c/IfeanyiOmeata
Linkedin: linkedin.com/in/omeatai
Twitter: twitter.com/iomeata
Github: github.com/omeatai
Stackoverflow: stackoverflow.com/users/2689166/omeatai
Hashnode: hashnode.com/@omeatai
Medium: medium.com/@omeatai
© 2021